Laravel Helpers... what now? Explain it in League terms please
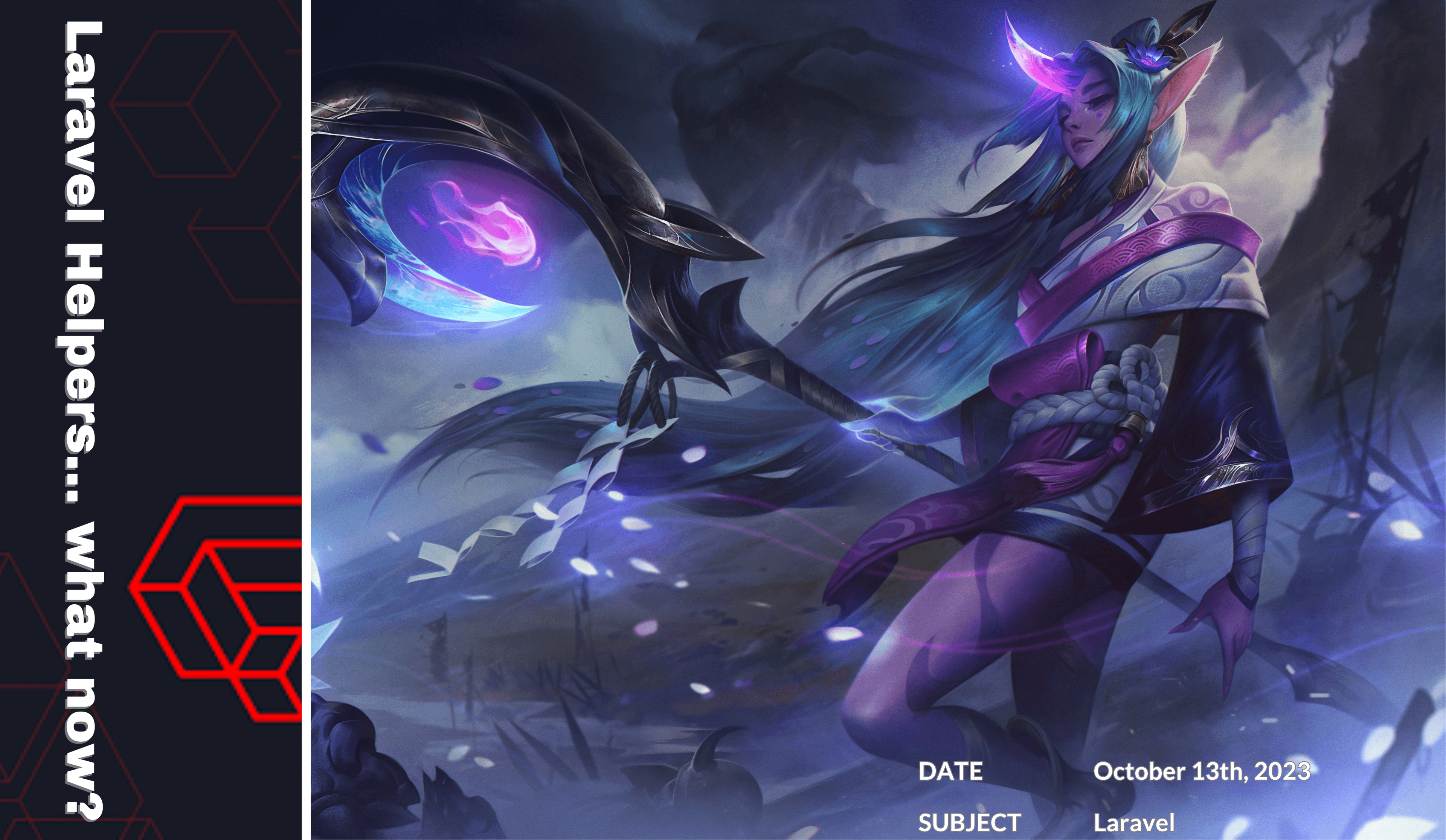
When developing web applications with Laravel, one of the many features that make the framework so powerful and developer-friendly is its rich set of helper functions. These small but mighty tools streamline common tasks, boost productivity, and enhance code readability. So basically it's like the support class is constantly healing you instead of getting in your way..
Why Helper Functions Matter
Helper functions in Laravel serve as utility tools, simplifying various coding tasks and making your application development process smoother. Whether you're working with arrays, strings, or performing various operations, Laravel's helpers are your trusty companions.
Practical Use Cases
In my current application that I'm working on for example, I've harnessed Laravel's helper functions to make my code cleaner and more efficient. Let's explore a few use cases with code examples:
$data = [
'user' => [
'name' => 'Safou',
'email' => 'safou@example.com',
],
];
$flattened = array_dot($data);
String manipulation is a common task. Laravel's str_contains() helps you check if a string contains a specific substring:
if (str_contains($content, 'video')) {
}
URL Helper Functions
<a href="{{ url('/profile') }}">Visit Profile</a>
Creating a Custom helpers.php File
In Laravel, you can create a custom helpers.php file to define your own helper functions. This file is typically stored in the app directory, and you need to autoload it by updating your composer.json file and running
composer dump-autoload.
Here's an example of a custom helpers.php file:
if (!function_exists('custom_function')) {
function custom_function() {
return 'This is a custom helper function!';
}
}
I for example have created function to handle some common taks that are specific to my applcation
Helper Functions for Genders and Roles
Let's say you have a gender selection feature in your application. You've defined constants for genders in a separate file, like Constants/Genders.php. In your helper functions, you can use these constants to make the code more readable:
../Constants/Genders.php
class Genders {
const MALE = 0;
const FEMALE = 1;
const PREFER_NOT_TO_SAY = 2;
}
helpers.php
if (!function_exists('get_gender_name')) {
function get_gender_name($gender) {
switch ($gender) {
case Genders::MALE:
return 'Male';
case Genders::FEMALE:
return 'Female';
case Genders::PREFER_NOT_TO_SAY:
return 'Prefer not to say';
default:
return 'Unknown';
}
}
}
This custom function get_gender_name() takes a gender constant as a parameter and returns the corresponding human-readable gender name.
Managing User Roles
Now let's say you have a role selection feature in your application. You've defined constants for roles in a separate file, like Constants/UserRoles.php. In your helper functions, you can use these constants to make the code more readable:
class UserRoles {
const DEFAULT_USER = 0;
const MODERATOR = 1;
const ADMIN = 2;
const OWNER = 4;
}
if (!function_exists('is_admin')) {
function isAdmin($userRole) {
return $userRole === UserRoles::ADMIN || $userRole === UserRoles::OWNER;
}
}
The isAdmin() function checks if a user has an admin role, which includes both the "Admin" and "Owner" roles.
So what are the benefits then
With these helper functions, you've made your code more readable and maintainable. For example, in a Blade view, you can use the get_gender_name() function to display the user's gender:
<span>{{ get_gender_name($user->gender) }}</span>
Similarly, I used the isAdmin() function in your controllers or views to determine if a user has admin privileges:
if (isaAmin($user->role)) {
// Perform admin-specific actions
}
By centralizing common logic in helper functions and using constants, our code becomes more concise and easier to understand, making it simpler to manage and maintain our Laravel application.
That is all for now we'll see each other soon bye!
Sources:
https://laravel.com/docs/10.x
https://laravel.com/docs/10.x/helpers#main-content
https://stackoverflow.com/questions/45004604/how-to-make-a-custom-helper-function-available-in-every-controller-for-laravel